The Building Blocks of a Node.js API
Application Program Interfaces (API) have become the building blocks of modern day applications and even these api's are built up of smaller components. When a node.js app is initialised or created a multitude of components are put together in the node_modules directory and any additional components downloaded are added in here as well.
In addition to this most api's need to be able to connect to other api's and databases, have some routing abilities, be able to schedule tasks, logging of its activities apart from the functionality that will be specific to them i.e. the reason they exist and these are not typically added by default primarily because these are really not needed by all api's.
NPM is seperate from node and is a package manager used to handle dependencies using the package.json file.
Express is a web application framework for Node.js, designed for building web applications and APIs. It provides a set of functions and middleware to create web servers and route HTTP requests to specific handling functions. Express is lightweight and flexible, and is a popular choice for building web applications with Node.js.
CORS (Cross-Origin Resource Sharing) is a security feature implemented by web browsers that blocks web pages from making requests to a different domain than the one that served the web page. This is done to prevent malicious websites from making unauthorized requests on behalf of the user.
For example, if a web page served from "example.com" tries to make a request to "api.example.com", the browser will block the request by default, unless "api.example.com" has explicitly allowed cross-origin requests from "example.com". This is done using HTTP headers, such as the "Access-Control-Allow-Origin" header, which can be set by the server to indicate which origins are allowed to make requests to the server.
CORS can be used to allow or block specific cross-origin requests, or to allow all cross-origin requests from any domain. It is an important security feature that helps to protect against cross-site request forgery (CSRF) attacks.
AXIOS is a JavaScript library that provides an easy-to-use interface for making HTTP requests. It can be used in web applications and Node.js applications to send HTTP requests to REST APIs, and to receive and process responses from these APIs.
Axios makes it easy to send HTTP requests from JavaScript, including requests that use the GET, POST, PUT, DELETE, and PATCH methods. It also provides features for handling responses, including automatic parsing of JSON data, and handling of HTTP errors.
Axios is a popular choice for making HTTP requests in JavaScript because it is easy to use, and provides a simple API that is similar to the fetch API, which is available in modern web browsers. It can be used in a variety of contexts, including making requests to REST APIs, and handling the response data in web applications and Node.js applications.
Here is an example of how you can use Axios to send a GET request to the GitHub API to retrieve a list of repositories for a particular user:
import axios from 'axios';
async function getRepos() {
try {
const response = await axios.get('https://api.github.com/users/USERNAME/repos');
console.log(response.data);
} catch (error) {
console.error(error);
}
}
getRepos();
In this example, the get
method is used to send a GET request to the GitHub API. The await
keyword is used to wait for the response before logging the data to the console. If there is an error, it will be caught by the catch
block and logged to the console.
Axios has a lot of other useful features, such as the ability to make POST, PUT, and DELETE requests, support for headers and authentication, and the ability to transform request and response data.
Nodemon Nodemon is a tool that automatically restarts a Node.js application when the source code is changed. It is commonly used during development to make it easier to test and debug an application, as it saves time by eliminating the need to manually stop and start the application each time a change is made.
To use Nodemon, you need to install it as a development dependency in your project using the following command:
npm install --save-dev nodemon
Once installed, you can use Nodemon to start your application by running the following command:
nodemon app.js
Here, app.js
is the entry point to your application. Nodemon will start the application and will automatically restart it whenever it detects a change in the source code.
You can also use Nodemon to run other scripts, such as test scripts, by specifying the script name as an argument:
nodemon test.js
To configure Nodemon in the package.json
file of your project, you can use the scripts
field to define a custom script that runs Nodemon with the desired options.
For example, let's say you want to use Nodemon to start your application when you run the start
script. You can do this by adding the following to your package.json
file:
{
"scripts": {
"start": "nodemon app.js"
}
}
Then, you can start your application using Nodemon by running the following command:
npm start
You can also specify additional options for Nodemon by adding them after the script name. For example, let's say you want to ignore the node_modules
directory and use the --inspect
flag to enable the Node.js debugger when running the start
script. You can do this by adding the following to your package.json
file:
{
"scripts": {
"start": "nodemon --ignore node_modules --inspect app.js"
}
}
This will start your application using Nodemon and will ignore any changes in the node_modules
directory. It will also enable the Node.js debugger, which allows you to debug your application using tools like the Chrome DevTools.
Nodemon has a number of additional options that can be used to customise its behavior. For example, you can use the --ignore
option to specify a list of files or directories that Nodemon should ignore when watching for changes. You can also use the --exec
option to specify a different interpreter to use when running the script.
You can read more about the available options for Nodemon in the documentation: https://github.com/remy/nodemon/blob/master/doc/cli/nodemon.md
Bring it all together
Create the directory
mkdir api
Initialise the npm application
npm init -y
Install the dependencies
npm install express cors axios nodemon
Create an index.js file, import packages and call the functions
const express = require('express');
const app = express();
app.listen(5000, () => {
console.log('API running on port 5000')
})
Update scripts section in package.json to use nodemon on start
{
"name": "api",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"start": "npx nodemon index.js"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"axios": "^1.1.3",
"cors": "^2.8.5",
"express": "^4.18.2",
"nodemon": "^2.0.20"
}
}
Run api in the terminal
npm start
This will run the api and listen on port 5000
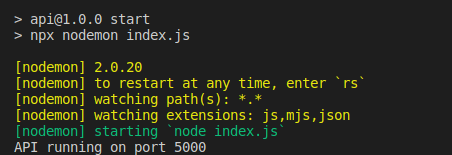
And there you have it. An api running on node.js from here you build out the rest of the application and things can really get interesting and complicated.