How To Structure Your Node Projects
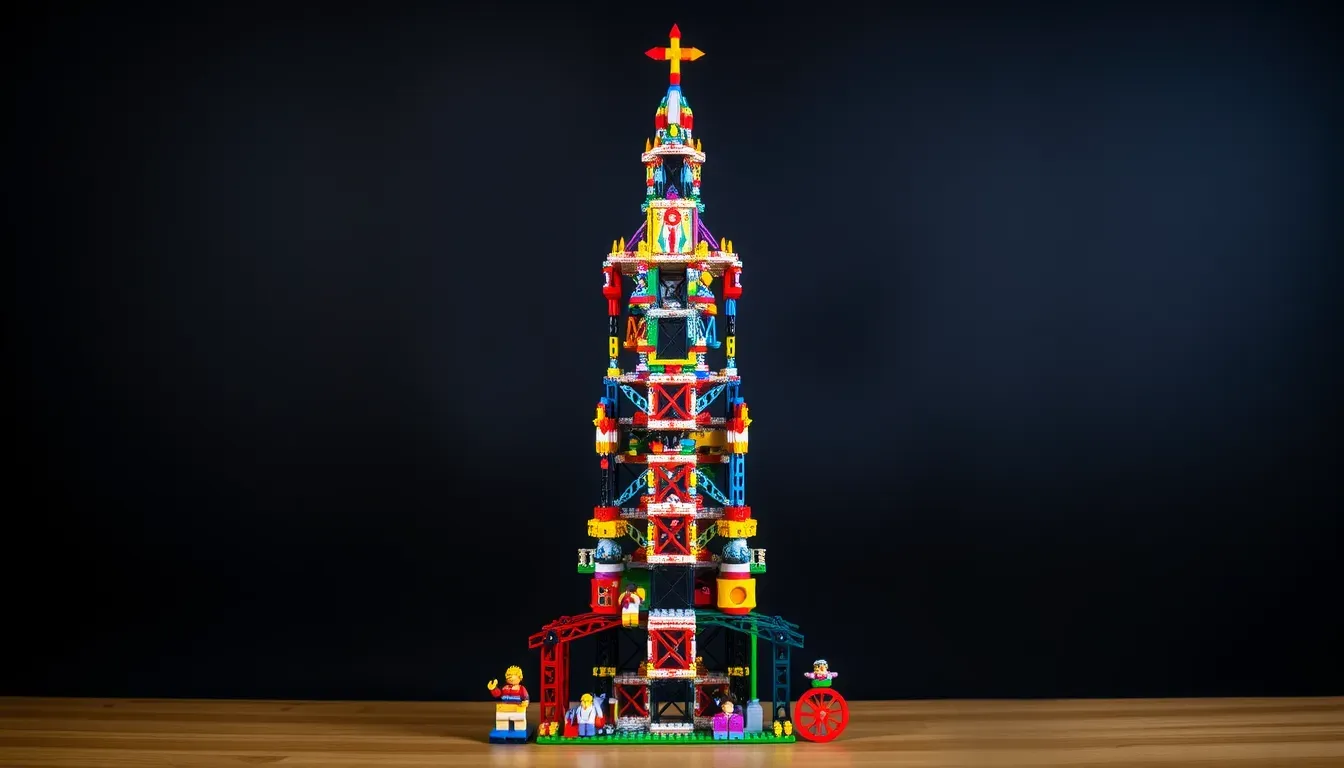
Structuring a Node.js application effectively is crucial for maintainability, scalability, and ease of development. Here are some best practices to consider:
1. Organize Your Code into Modules
- Modular Code: Break your code into smaller, manageable modules. This helps in maintaining a clean and organized codebase.
2. Use the MVC Pattern
- Model-View-Controller (MVC): This design pattern separates your application into three interconnected components. It helps in organizing your code and separating concerns.
3. Environment Variables for Configuration
- Configuration Management: Use environment variables to manage configuration settings. This keeps sensitive information out of your codebase.
4. Middleware for Common Functionality
- Middleware: Use middleware to handle common functionalities like authentication, logging, and error handling.
5. Layered Approach
- Layered Architecture: Implement a layered approach to separate different parts of your application, such as services, controllers, and data access layers.
6. Folder Structure
- Organized Folders: Use a clear and consistent folder structure. Common directories include
models
,views
,controllers
,services
,config
, and `routes.
7. Code Readability and Clean Code
- Clean Code: Focus on writing clean, readable code. Use linters and follow a style guide to maintain consistency.
8. Asynchronous Code
- Async/Await: Write asynchronous code using promises and
async/await
to handle asynchronous operations efficiently.
9. Testing
- Unit Testing: Conduct unit tests to ensure your code works as expected. This helps in catching bugs early and maintaining code quality.
10. Error Handling
- Error Handling: Implement robust error handling to manage unexpected issues gracefully.
By following these best practices, you can build a Node.js application that is clean, maintainable, and scalable.
Here’s an example of how you might structure a Node.js application following the best practices mentioned:
Project Structure
my-node-app/
├── config/
│ ├── config.js
├── controllers/
│ ├── userController.js
├── models/
│ ├── userModel.js
├── routes/
│ ├── userRoutes.js
├── services/
│ ├── userService.js
├── middlewares/
│ ├── authMiddleware.js
├── views/
│ ├── index.ejs
├── tests/
│ ├── userController.test.js
├── .env
├── app.js
├── package.json
└── README.md
Example Files
config/config.js
module.exports = {
db: process.env.DB_CONNECTION_STRING,
port: process.env.PORT || 3000,
};
models/userModel.js
const mongoose = require('mongoose');
const userSchema = new mongoose.Schema({
name: String,
email: String,
password: String,
});
module.exports = mongoose.model('User', userSchema);
controllers/userController.js
const UserService = require('../services/userService');
exports.getUser = async (req, res) => {
try {
const user = await UserService.getUserById(req.params.id);
res.json(user);
} catch (error) {
res.status(500).send(error.message);
}
};
services/userService.js
const User = require('../models/userModel');
exports.getUserById = async (id) => {
return await User.findById(id);
};
routes/userRoutes.js
const express = require('express');
const router = express.Router();
const UserController = require('../controllers/userController');
router.get('/user/:id', UserController.getUser);
module.exports = router;
middlewares/authMiddleware.js
module.exports = (req, res, next) => {
// Authentication logic here
next();
};
app.js
const express = require('express');
const mongoose = require('mongoose');
const config = require('./config/config');
const userRoutes = require('./routes/userRoutes');
const app = express();
mongoose.connect(config.db, { useNewUrlParser: true, useUnifiedTopology: true });
app.use(express.json());
app.use('/api', userRoutes);
app.listen(config.port, () => {
console.log(`Server running on port ${config.port}`);
});
This structure helps keep your code organized and modular, making it easier to manage and scale.