Basic Node.js Test With Mocha
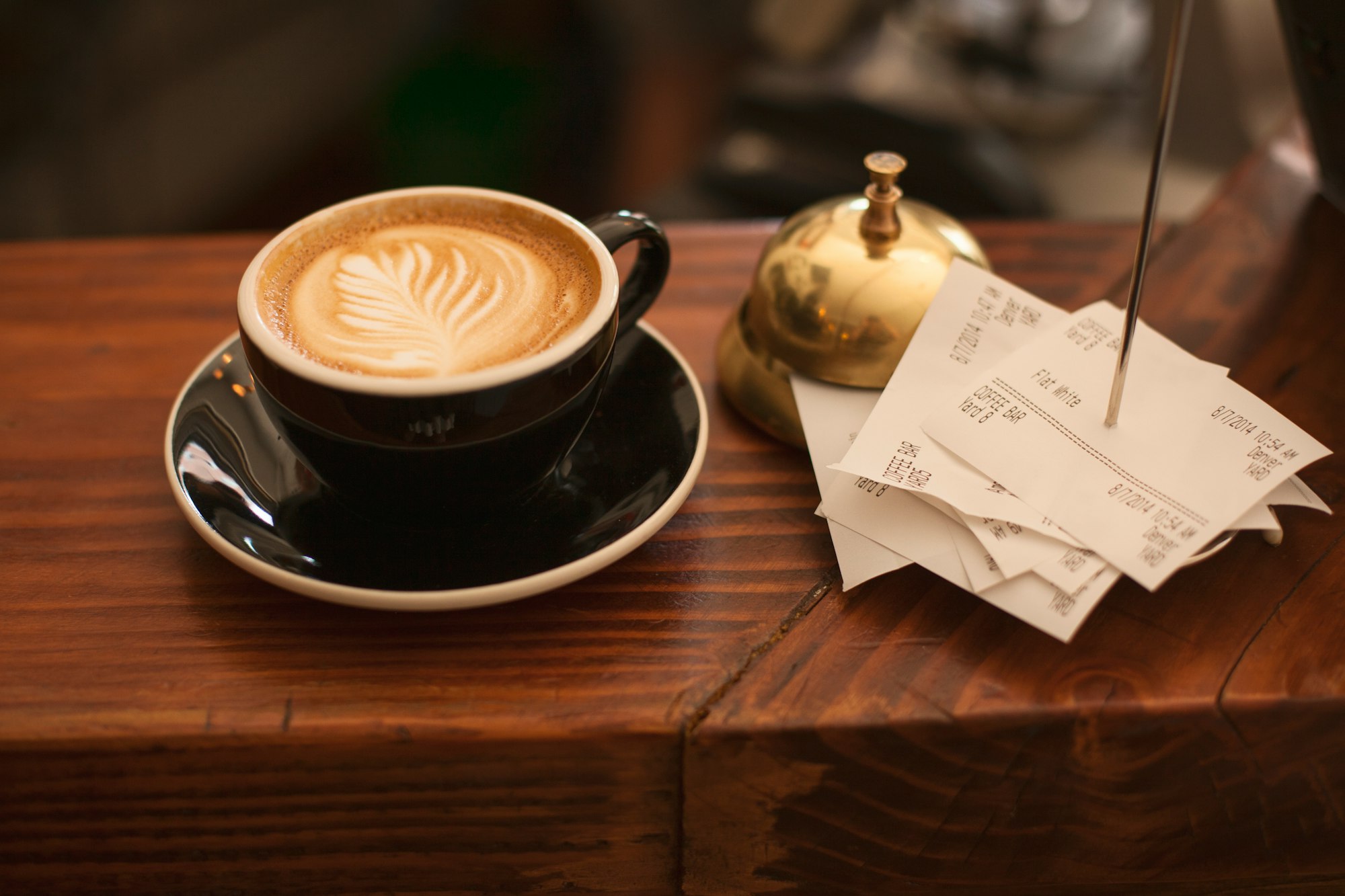
Here is an example of a test written in Mocha, a popular JavaScript testing framework for Node.js:
const assert = require('assert');
describe('my test', () => {
it('should run a test', () => {
const x = 1;
const y = 1;
assert.equal(x, y);
});
});
This code defines a test using Mocha's describe
and it
functions. The describe
function is used to group tests together, and the it
function is used to define an individual test.
The test itself uses the assert.equal
function from the assert
module to check that the values of x
and y
are equal. If they are not equal, the test will fail.
To run this test, you would use the mocha
command in your terminal, followed by the name of the file containing the test:
$ mocha my-test.js
This will run the test and print the results to the screen. If the test passes, you should see something like this:
my test
√ should run a test
1 passing (10ms)
If the test fails, you will see an error message indicating why the test failed.
That's it.. Well the basics anyway.